35 Python Programming Exercises and Solutions
To understand a programming language deeply, you need to practice what you’ve learned. If you’ve completed learning the syntax of Python programming language, it is the right time to do some practice programs.

1. Python program to check whether the given number is even or not.
2. python program to convert the temperature in degree centigrade to fahrenheit, 3. python program to find the area of a triangle whose sides are given, 4. python program to find out the average of a set of integers, 5. python program to find the product of a set of real numbers, 6. python program to find the circumference and area of a circle with a given radius, 7. python program to check whether the given integer is a multiple of 5, 8. python program to check whether the given integer is a multiple of both 5 and 7, 9. python program to find the average of 10 numbers using while loop, 10. python program to display the given integer in a reverse manner, 11. python program to find the geometric mean of n numbers, 12. python program to find the sum of the digits of an integer using a while loop, 13. python program to display all the multiples of 3 within the range 10 to 50, 14. python program to display all integers within the range 100-200 whose sum of digits is an even number, 15. python program to check whether the given integer is a prime number or not, 16. python program to generate the prime numbers from 1 to n, 17. python program to find the roots of a quadratic equation, 18. python program to print the numbers from a given number n till 0 using recursion, 19. python program to find the factorial of a number using recursion, 20. python program to display the sum of n numbers using a list, 21. python program to implement linear search, 22. python program to implement binary search, 23. python program to find the odd numbers in an array, 24. python program to find the largest number in a list without using built-in functions, 25. python program to insert a number to any position in a list, 26. python program to delete an element from a list by index, 27. python program to check whether a string is palindrome or not, 28. python program to implement matrix addition, 29. python program to implement matrix multiplication, 30. python program to check leap year, 31. python program to find the nth term in a fibonacci series using recursion, 32. python program to print fibonacci series using iteration, 33. python program to print all the items in a dictionary, 34. python program to implement a calculator to do basic operations, 35. python program to draw a circle of squares using turtle.
For practicing more such exercises, I suggest you go to hackerrank.com and sign up. You’ll be able to practice Python there very effectively.
I hope these exercises were helpful to you. If you have any doubts, feel free to let me know in the comments.
12 thoughts on “ 35 Python Programming Exercises and Solutions ”
I don’t mean to nitpick and I don’t want this published but you might want to check code for #16. 4 is not a prime number.
You can only check if integer is a multiple of 35. It always works the same – just multiply all the numbers you need to check for multiplicity.
v_str = str ( input(‘ Enter a valid string or number :- ‘) ) v_rev_str=” for v_d in v_str: v_rev_str = v_d + v_rev_str
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Recent Posts
Python Programming
Practice Python Exercises and Challenges with Solutions
Free Coding Exercises for Python Developers. Exercises cover Python Basics , Data structure , to Data analytics . As of now, this page contains 18 Exercises.
What included in these Python Exercises?
Each exercise contains specific Python topic questions you need to practice and solve. These free exercises are nothing but Python assignments for the practice where you need to solve different programs and challenges.
- All exercises are tested on Python 3.
- Each exercise has 10-20 Questions.
- The solution is provided for every question.
- Practice each Exercise in Online Code Editor
These Python programming exercises are suitable for all Python developers. If you are a beginner, you will have a better understanding of Python after solving these exercises. Below is the list of exercises.
Select the exercise you want to solve .
Basic Exercise for Beginners
Practice and Quickly learn Python’s necessary skills by solving simple questions and problems.
Topics : Variables, Operators, Loops, String, Numbers, List
Python Input and Output Exercise
Solve input and output operations in Python. Also, we practice file handling.
Topics : print() and input() , File I/O
Python Loop Exercise
This Python loop exercise aims to help developers to practice branching and Looping techniques in Python.
Topics : If-else statements, loop, and while loop.
Python Functions Exercise
Practice how to create a function, nested functions, and use the function arguments effectively in Python by solving different questions.
Topics : Functions arguments, built-in functions.
Python String Exercise
Solve Python String exercise to learn and practice String operations and manipulations.
Python Data Structure Exercise
Practice widely used Python types such as List, Set, Dictionary, and Tuple operations in Python
Python List Exercise
This Python list exercise aims to help Python developers to learn and practice list operations.
Python Dictionary Exercise
This Python dictionary exercise aims to help Python developers to learn and practice dictionary operations.
Python Set Exercise
This exercise aims to help Python developers to learn and practice set operations.
Python Tuple Exercise
This exercise aims to help Python developers to learn and practice tuple operations.
Python Date and Time Exercise
This exercise aims to help Python developers to learn and practice DateTime and timestamp questions and problems.
Topics : Date, time, DateTime, Calendar.
Python OOP Exercise
This Python Object-oriented programming (OOP) exercise aims to help Python developers to learn and practice OOP concepts.
Topics : Object, Classes, Inheritance
Python JSON Exercise
Practice and Learn JSON creation, manipulation, Encoding, Decoding, and parsing using Python
Python NumPy Exercise
Practice NumPy questions such as Array manipulations, numeric ranges, Slicing, indexing, Searching, Sorting, and splitting, and more.
Python Pandas Exercise
Practice Data Analysis using Python Pandas. Practice Data-frame, Data selection, group-by, Series, sorting, searching, and statistics.
Python Matplotlib Exercise
Practice Data visualization using Python Matplotlib. Line plot, Style properties, multi-line plot, scatter plot, bar chart, histogram, Pie chart, Subplot, stack plot.
Random Data Generation Exercise
Practice and Learn the various techniques to generate random data in Python.
Topics : random module, secrets module, UUID module
Python Database Exercise
Practice Python database programming skills by solving the questions step by step.
Use any of the MySQL, PostgreSQL, SQLite to solve the exercise
Exercises for Intermediate developers
The following practice questions are for intermediate Python developers.
If you have not solved the above exercises, please complete them to understand and practice each topic in detail. After that, you can solve the below questions quickly.
Exercise 1: Reverse each word of a string
Expected Output
- Use the split() method to split a string into a list of words.
- Reverse each word from a list
- finally, use the join() function to convert a list into a string
Steps to solve this question :
- Split the given string into a list of words using the split() method
- Use a list comprehension to create a new list by reversing each word from a list.
- Use the join() function to convert the new list into a string
- Display the resultant string
Exercise 2: Read text file into a variable and replace all newlines with space
Given : Assume you have a following text file (sample.txt).
Expected Output :
- First, read a text file.
- Next, use string replace() function to replace all newlines ( \n ) with space ( ' ' ).
Steps to solve this question : -
- First, open the file in a read mode
- Next, read all content from a file using the read() function and assign it to a variable.
- Display final string
Exercise 3: Remove items from a list while iterating
Description :
In this question, You need to remove items from a list while iterating but without creating a different copy of a list.
Remove numbers greater than 50
Expected Output : -
- Get the list's size
- Iterate list using while loop
- Check if the number is greater than 50
- If yes, delete the item using a del keyword
- Reduce the list size
Solution 1: Using while loop
Solution 2: Using for loop and range()
Exercise 4: Reverse Dictionary mapping
Exercise 5: display all duplicate items from a list.
- Use the counter() method of the collection module.
- Create a dictionary that will maintain the count of each item of a list. Next, Fetch all keys whose value is greater than 2
Solution 1 : - Using collections.Counter()
Solution 2 : -
Exercise 6: Filter dictionary to contain keys present in the given list
Exercise 7: print the following number pattern.
Refer to Print patterns in Python to solve this question.
- Use two for loops
- The outer loop is reverse for loop from 5 to 0
- Increment value of x by 1 in each iteration of an outer loop
- The inner loop will iterate from 0 to the value of i of the outer loop
- Print value of x in each iteration of an inner loop
- Print newline at the end of each outer loop
Exercise 8: Create an inner function
Question description : -
- Create an outer function that will accept two strings, x and y . ( x= 'Emma' and y = 'Kelly' .
- Create an inner function inside an outer function that will concatenate x and y.
- At last, an outer function will join the word 'developer' to it.
Exercise 9: Modify the element of a nested list inside the following list
Change the element 35 to 3500
Exercise 10: Access the nested key increment from the following dictionary
Under Exercises: -
Python Object-Oriented Programming (OOP) Exercise: Classes and Objects Exercises
Updated on: December 8, 2021 | 52 Comments
Python Date and Time Exercise with Solutions
Updated on: December 8, 2021 | 10 Comments
Python Dictionary Exercise with Solutions
Updated on: May 6, 2023 | 56 Comments
Python Tuple Exercise with Solutions
Updated on: December 8, 2021 | 96 Comments
Python Set Exercise with Solutions
Updated on: October 20, 2022 | 27 Comments
Python if else, for loop, and range() Exercises with Solutions
Updated on: September 3, 2024 | 298 Comments
Updated on: August 2, 2022 | 155 Comments
Updated on: September 6, 2021 | 109 Comments
Python List Exercise with Solutions
Updated on: December 8, 2021 | 201 Comments
Updated on: December 8, 2021 | 7 Comments
Python Data Structure Exercise for Beginners
Updated on: December 8, 2021 | 116 Comments
Python String Exercise with Solutions
Updated on: October 6, 2021 | 221 Comments
Updated on: March 9, 2021 | 23 Comments
Updated on: March 9, 2021 | 51 Comments
Updated on: July 20, 2021 | 29 Comments
Python Basic Exercise for Beginners
Updated on: August 29, 2024 | 498 Comments
Useful Python Tips and Tricks Every Programmer Should Know
Updated on: May 17, 2021 | 23 Comments
Python random Data generation Exercise
Updated on: December 8, 2021 | 13 Comments
Python Database Programming Exercise
Updated on: March 9, 2021 | 17 Comments
- Online Python Code Editor
Updated on: June 1, 2022 |
About PYnative
PYnative.com is for Python lovers. Here, You can get Tutorials, Exercises, and Quizzes to practice and improve your Python skills .
Explore Python
- Learn Python
- Python Basics
- Python Databases
- Python Exercises
- Python Quizzes
- Python Tricks
To get New Python Tutorials, Exercises, and Quizzes
Legal Stuff
We use cookies to improve your experience. While using PYnative, you agree to have read and accepted our Terms Of Use , Cookie Policy , and Privacy Policy .
Copyright © 2018–2024 pynative.com
Interested in a verified certificate or a professional certificate ?
An introduction to programming using a language called Python. Learn how to read and write code as well as how to test and “debug” it. Designed for students with or without prior programming experience who’d like to learn Python specifically. Learn about functions, arguments, and return values (oh my!); variables and types; conditionals and Boolean expressions; and loops. Learn how to handle exceptions, find and fix bugs, and write unit tests; use third-party libraries; validate and extract data with regular expressions; model real-world entities with classes, objects, methods, and properties; and read and write files. Hands-on opportunities for lots of practice. Exercises inspired by real-world programming problems. No software required except for a web browser, or you can write code on your own PC or Mac.
Whereas CS50x itself focuses on computer science more generally as well as programming with C, Python, SQL, and JavaScript, this course, aka CS50P, is entirely focused on programming with Python. You can take CS50P before CS50x, during CS50x, or after CS50x. But for an introduction to computer science itself, you should still take CS50x!
How to Take this Course
Even if you are not a student at Harvard, you are welcome to “take” this course for free via this OpenCourseWare by working your way through the course’s ten weeks of material. For each week, follow this workflow:
And then submit the course’s final project .
To submit the course’s problem sets and final project for feedback, be sure to create an edX account , if you haven’t already. Ask questions along the way via any of the course’s communities !
- If interested in a verified certificate from edX , enroll at cs50.edx.org/python instead.
- If interested in a professional certificate from edX , enroll at cs50.edx.org/programs/python (for Python) or cs50.edx.org/programs/data (for Data Science) instead.
How to Teach this Course
If you are a teacher, you are welcome to adopt or adapt these materials for your own course, per the license .
Browse Course Material
Course info, instructors.
- Dr. Ana Bell
- Prof. Eric Grimson
- Prof. John Guttag
Departments
- Electrical Engineering and Computer Science
As Taught In
- Algorithms and Data Structures
- Programming Languages
Learning Resource Types
Introduction to computer science and programming in python, course description.
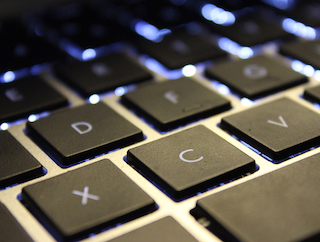
You are leaving MIT OpenCourseWare
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively.
Python Examples
The best way to learn Python is by practicing examples. This page contains examples on basic concepts of Python. We encourage you to try these examples on your own before looking at the solution.
All the programs on this page are tested and should work on all platforms.
Want to learn Python by writing code yourself? Enroll in our Interactive Python Course for FREE.
- Python Program to Check Prime Number
- Python Program to Add Two Numbers
- Python Program to Find the Factorial of a Number
- Python Program to Make a Simple Calculator
- Python Program to Print Hello world!
- Python Program to Find the Square Root
- Python Program to Calculate the Area of a Triangle
- Python Program to Solve Quadratic Equation
- Python Program to Swap Two Variables
- Python Program to Generate a Random Number
- Python Program to Convert Kilometers to Miles
- Python Program to Convert Celsius To Fahrenheit
- Python Program to Check if a Number is Positive, Negative or 0
- Python Program to Check if a Number is Odd or Even
- Python Program to Check Leap Year
- Python Program to Find the Largest Among Three Numbers
- Python Program to Print all Prime Numbers in an Interval
- Python Program to Display the multiplication Table
- Python Program to Print the Fibonacci sequence
- Python Program to Check Armstrong Number
- Python Program to Find Armstrong Number in an Interval
- Python Program to Find the Sum of Natural Numbers
- Python Program to Display Powers of 2 Using Anonymous Function
- Python Program to Find Numbers Divisible by Another Number
- Python Program to Convert Decimal to Binary, Octal and Hexadecimal
- Python Program to Find ASCII Value of Character
- Python Program to Find HCF or GCD
- Python Program to Find LCM
- Python Program to Find the Factors of a Number
- Python Program to Shuffle Deck of Cards
- Python Program to Display Calendar
- Python Program to Display Fibonacci Sequence Using Recursion
- Python Program to Find Sum of Natural Numbers Using Recursion
- Python Program to Find Factorial of Number Using Recursion
- Python Program to Convert Decimal to Binary Using Recursion
- Python Program to Add Two Matrices
- Python Program to Transpose a Matrix
- Python Program to Multiply Two Matrices
- Python Program to Check Whether a String is Palindrome or Not
- Python Program to Remove Punctuations From a String
- Python Program to Sort Words in Alphabetic Order
- Python Program to Illustrate Different Set Operations
- Python Program to Count the Number of Each Vowel
- Python Program to Merge Mails
- Python Program to Find the Size (Resolution) of an Image
- Python Program to Find Hash of File
- Python Program to Create Pyramid Patterns
- Python Program to Merge Two Dictionaries
- Python Program to Safely Create a Nested Directory
- Python Program to Access Index of a List Using for Loop
- Python Program to Flatten a Nested List
- Python Program to Slice Lists
- Python Program to Iterate Over Dictionaries Using for Loop
- Python Program to Sort a Dictionary by Value
- Python Program to Check If a List is Empty
- Python Program to Catch Multiple Exceptions in One Line
- Python Program to Copy a File
- Python Program to Concatenate Two Lists
- Python Program to Check if a Key is Already Present in a Dictionary
- Python Program to Split a List Into Evenly Sized Chunks
- Python Program to Parse a String to a Float or Int
- Python Program to Print Colored Text to the Terminal
- Python Program to Convert String to Datetime
- Python Program to Get the Last Element of the List
- Python Program to Get a Substring of a String
- Python Program to Print Output Without a Newline
- Python Program Read a File Line by Line Into a List
- Python Program to Randomly Select an Element From the List
- Python Program to Check If a String Is a Number (Float)
- Python Program to Count the Occurrence of an Item in a List
- Python Program to Append to a File
- Python Program to Delete an Element From a Dictionary
- Python Program to Create a Long Multiline String
- Python Program to Extract Extension From the File Name
- Python Program to Measure the Elapsed Time in Python
- Python Program to Get the Class Name of an Instance
- Python Program to Convert Two Lists Into a Dictionary
- Python Program to Differentiate Between type() and isinstance()
- Python Program to Trim Whitespace From a String
- Python Program to Get the File Name From the File Path
- Python Program to Represent enum
- Python Program to Return Multiple Values From a Function
- Python Program to Get Line Count of a File
- Python Program to Find All File with .txt Extension Present Inside a Directory
- Python Program to Get File Creation and Modification Date
- Python Program to Get the Full Path of the Current Working Directory
- Python Program to Iterate Through Two Lists in Parallel
- Python Program to Check the File Size
- Python Program to Reverse a Number
- Python Program to Compute the Power of a Number
- Python Program to Count the Number of Digits Present In a Number
- Python Program to Check If Two Strings are Anagram
- Python Program to Capitalize the First Character of a String
- Python Program to Compute all the Permutation of the String
- Python Program to Create a Countdown Timer
- Python Program to Count the Number of Occurrence of a Character in String
- Python Program to Remove Duplicate Element From a List
- Python Program to Convert Bytes to a String
- ▼Java Exercises
- ▼Java Basics
- Basic Part-I
- Basic Part-II
- ▼Java Data Types
- Java Enum Types
- ▼Java Control Flow
- Conditional Statement
- Recursive Methods
- ▼Java Math and Numbers
- ▼Object Oriented Programming
- Java Constructor
- Java Static Members
- Java Nested Classes
- Java Inheritance
- Java Abstract Classes
- Java Interface
- Java Encapsulation
- Java Polymorphism
- Object-Oriented Programming
- ▼Exception Handling
- Exception Handling Home
- ▼Functional Programming
- Java Lambda expression
- ▼Multithreading
- Java Thread
- Java Multithreading
- ▼Data Structures
- ▼Strings and I/O
- File Input-Output
- ▼Date and Time
- ▼Advanced Concepts
- Java Generic Method
- ▼Algorithms
- ▼Regular Expressions
- Regular Expression Home
- ▼JavaFx Exercises
- JavaFx Exercises Home
- ..More to come..
Java Programming Exercises, Practice, Solution
Java exercises.
Java is the foundation for virtually every type of networked application and is the global standard for developing and delivering embedded and mobile applications, games, Web-based content, and enterprise software. With more than 9 million developers worldwide, Java enables you to efficiently develop, deploy and use exciting applications and services.
The best way we learn anything is by practice and exercise questions. Here you have the opportunity to practice the Java programming language concepts by solving the exercises starting from basic to more complex exercises. A sample solution is provided for each exercise. It is recommended to do these exercises by yourself first before checking the solution.
Hope, these exercises help you to improve your Java programming coding skills. Currently, following sections are available, we are working hard to add more exercises .... Happy Coding!
List of Java Exercises:
- Basic Exercises Part-I [ 150 Exercises with Solution ]
- Basic Exercises Part-II [ 99 Exercises with Solution ]
- Methods [ 23 Exercises with Solution ]
- Data Types Exercises [ 15 Exercises with Solution ]
- Java Enum Types Exercises [ 5 Exercises with Solution ]
- Conditional Statement Exercises [ 32 Exercises with Solution ]
- Java recursive method Exercises [ 15 Exercises with Solution ]
- Math [ 27 Exercises with Solution ]
- Numbers [ 28 Exercises with Solution ]
- Java Constructor Exercises [ 10 exercises with solution ]
- Java Static Members Exercises [ 8 exercises with solution ]
- Java Nested Classes Exercises [ 10 exercises with solution ]
- Java Inheritance Exercises [ 9 exercises with solution ]
- Java Abstract Classes Exercises [ 12 exercises with solution ]
- Java Interface Exercises [ 11 exercises with solution ]
- Java Encapsulation Exercises [ 14 exercises with solution ]
- Java Polymorphism Exercises [ 12 exercises with solution ]
- Object-Oriented Programming [ 30 Exercises with Solution ]
- Exercises on handling and managing exceptions in Java [ 7 Exercises with Solution ]
- Java Lambda expression Exercises [ 25 exercises with solution ]
- Streams [ 8 Exercises with Solution ]
- Java Thread Exercises [ 7 exercises with solution ]
- Java Miltithreading Exercises [ 10 exercises with solution ]
- Array [ 77 Exercises with Solution ]
- Stack [ 29 Exercises with Solution ]
- Collection [ 126 Exercises with Solution ]
- String [ 107 Exercises with Solution ]
- Input-Output-File-System [ 18 Exercises with Solution ]
- Date Time [ 44 Exercises with Solution ]
- Java Generic Methods [ 7 exercises with solution ]
- Java Unit Test [ 10 Exercises with Solution ]
- Search [ 7 Exercises with Solution ]
- Sorting [ 19 Exercises with Solution ]
- Regular Expression [ 30 Exercises with Solution ]
- JavaFX [ 70 Exercises with Solution ]
Note: If you are not habituated with Java programming you can learn from the following :
- Java Programming Language
More to Come !
List of Exercises with Solutions :
- HTML CSS Exercises, Practice, Solution
- JavaScript Exercises, Practice, Solution
- jQuery Exercises, Practice, Solution
- jQuery-UI Exercises, Practice, Solution
- CoffeeScript Exercises, Practice, Solution
- Twitter Bootstrap Exercises, Practice, Solution
- C Programming Exercises, Practice, Solution
- C# Sharp Programming Exercises, Practice, Solution
- PHP Exercises, Practice, Solution
- Python Exercises, Practice, Solution
- R Programming Exercises, Practice, Solution
- Java Exercises, Practice, Solution
- SQL Exercises, Practice, Solution
- MySQL Exercises, Practice, Solution
- PostgreSQL Exercises, Practice, Solution
- SQLite Exercises, Practice, Solution
- MongoDB Exercises, Practice, Solution
[ Want to contribute to Java exercises? Send your code (attached with a .zip file) to us at w3resource[at]yahoo[dot]com. Please avoid copyrighted materials.]
Do not submit any solution of the above exercises at here, if you want to contribute go to the appropriate exercise page.
Follow us on Facebook and Twitter for latest update.
- Weekly Trends and Language Statistics
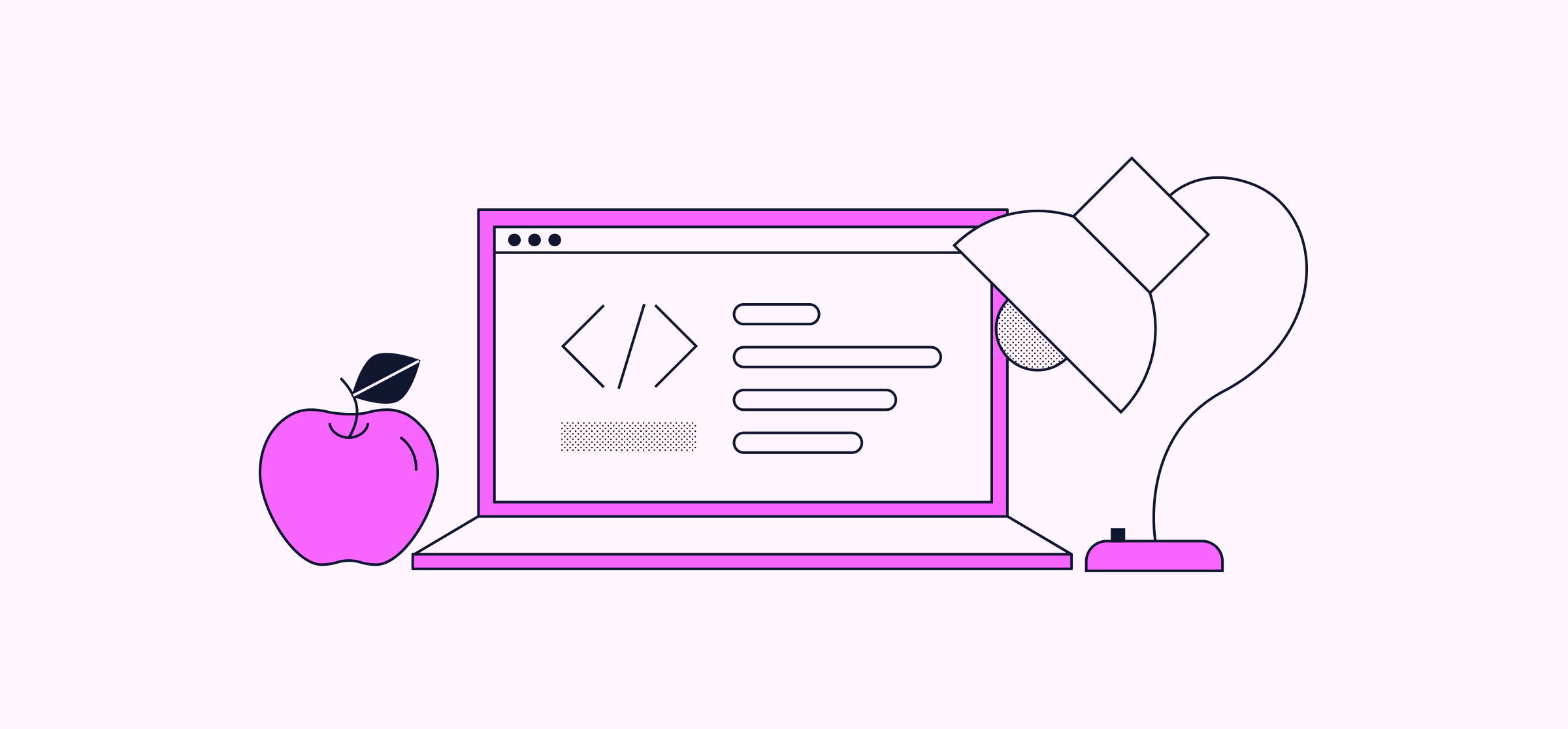
10 Coding Projects for Beginners
- Share article on Twitter
- Share article on Facebook
- Share article on LinkedIn
Learning to code is a satisfying, rewarding experience — especially if you’re teaching yourself with online programming courses . Still, most developers agree that if you really want to learn how to code, you’ll need to create something. What’s the point in learning about programming languages, libraries, and tools if you’re not applying that knowledge to a project?
Creating coding projects, like simple text-based applications, is the best way to instill the skills and knowledge you gain as you learn how to code. These projects help teach you the basics of programming, force you to think like a developer, and expose you to the tools you’ll use later in your career. To help you gain some hands-on experience, we’ve created this list of 10 coding projects for beginners.
Learn something new for free
- Intro to ChatGPT
How to begin coding
Before you tackle any of the projects listed below, you’ll need to learn how to code. But which programming language should you learn first? The answer depends on what you want to do with it.
If you want to build your own websites, you’ll need to learn programming languages like HTML, CSS , and JavaScript . If you’re more interested in scientific computing, languages like Python , C++ , or Java might be right for you.
To start learning any of these programming languages, check out the courses below:
- Learn JavaScript
- Learn Python
Once you’ve mastered your language of choice, put your skills to the test with the following projects.
10 coding projects for beginners
The following list of projects is designed to appeal to all skill levels, from new to experienced developers. Each project will teach you how to think like a programmer and build your skills with your languages, libraries, and other tools. You can also publish them on sites like GitHub to showcase your abilities. Here are 10 basic coding projects for beginners :
1. Build a chess game
Building a chess game is a great way to hone your ability to think like a developer. It’ll also allow you to practice using algorithms, as you’ll have to create not only the board and game pieces but also the specific moves that each piece can make.
2. Make a mobile app
Learning how to build mobile applications is an excellent choice if you’re looking to break into mobile development . Depending on your preference of platform, you might have to learn either Swift (for iOS apps) or Kotlin (for Android apps).
Need some guidance as you build your first mobile app? Check out either of the Skill Paths below:
- Build Basic Android Apps with Java
- Build iOS Apps with SwiftUI
3. Create a basic calculator
Building a calculator is a popular project for new developers, as you’ll need to create both a layout and an algorithm that can process numbers and symbols. While it may seem simple, you’ll have to closely examine your code and process.
4. Build a web scraper
In this project, you’ll use Python and libraries like Beautiful Soup to extract information from HTML and XML files and pages. This is great practice if you’re considering a career in data science . If you need help with this project, try our web scraping with Beautiful Soup course .
5. Create a Javascript slideshow
While this project doesn’t involve tons of work, it is important. It teaches you how to use a Document Object Model (DOM) in a web browser to make a website dynamic. If you’re pursuing a career in web development , this will be one of your many responsibilities.
What’s great about this type of project is that once you know how to create a JavaScript Slideshow, you can apply it to various websites with different designs.
6. Make a countdown timer
A countdown timer tracks the years, months, days, hours, and seconds until an event occurs. This project tests your ability to create a date field, optional time, and a start button. Once it’s complete, you’ll be able to count down the time until any event you have in mind.
7. Flip images
As a developer, you’ll likely find yourself working with digital images at some point in your career. Learning how to alter, resize, and flip them will give you an edge over the competition. For this project, all you need is HTML, CSS, and JavaScript.
8. Develop a recipe app
If you like to cook but have trouble organizing your recipes, creating a recipe app could be a fun and useful project. You’ll want the app to list your recipes by title, displaying a recipe card and picture. You’ll also want to add information about serving sizes, difficulty level, ingredients, and preparation.
9. Create a book finder app
If you’re a book lover with a large library of uncategorized books, a book finder app could be a great assistant — especially if you want to learn more about a book and its author. With this type of app, you’ll need to create a search field that returns relevant information about a book.
10. Build a drawing app
If you have a passion for the visual arts and long for a digital drawing space, try building your own. Within the app, you should be able to draw images with your cursor, manipulate colors, draw and alter shapes, and save the drawing to a local device. Bonus points if you make the images shareable.
Portfolio Projects
Along with the 10 listed above, there’s an almost endless amount of other projects that you can complete to build and showcase your technical skills. If you need help finding one, check out our Portfolio Projects, found in each of our Career Paths.
Our Career Paths are designed to help you learn the skills you’ll need to land an entry-level position in the tech industry. As you complete your Path, you’ll use the skills and knowledge you’ve learned to create various projects that’ll help you illustrate your skills to potential employers.
- Front-End Engineer Career Path : Learn front-end languages and frameworks and use them to create a custom Spotify playlist, add animations to static web pages, and more.
- Back-End Engineer Career Path : Learn back-end development with tools like SQL, Express, and PostgreSQL, and use them to build a comic book company API from scratch.
- Full-Stack Software Engineer Career Path: Learn both front-end and back-end development as you create a database for a restaurant’s menu.
- Computer Science Career Path : Master Python and development tools like Command Line and Git as you learn how to create your own interactive Choose Your Own Adventure game.
- Data Scientist Career Path : Venture into data science, database management, machine learning, and more while learning how to visualize your data.
- Data Analyst Career Path : Explore Python, SQL, and the tools you’ll need to analyze data and use them to visualize data pulled from the World Cup.
Whether you’re looking to break into a new career, build your technical skills, or just code for fun, we’re here to help every step of the way. Check out our blog post about how to choose the best Codecademy plan for you to learn about our structured courses, professional certifications, interview prep resources, career services, and more.
Related courses
Learn to code with blockly, choosing a programming language, choosing a career in tech, subscribe for news, tips, and more, related articles.

Behind the Build: Designing Post-Quiz Review
Feedback is back in town with post-quiz review.

How to Estimate the Amount of Time You Need for a Project
How long is a piece of string? Estimating software engineering work is part science, part finger in the air — here’s some practical advice to get started.

How to Use AI to Get Ahead in School
Get AI-ready for the school year by learning these concepts.

8 Ways Students Use Codecademy to Excel in Class (& Life)
Learn the skills you’ll actually use in the real world with Codecademy Student Pro.

7 Small Wins To Celebrate On Your Journey To Becoming A Professional Developer
Having an end goal is important, but so is celebrating your progress. Here are some milestones to look forward to as you learn how to code.

7 Most Popular Programming Languages for Game Development
Learn the best languages for game development and why developers choose to use them. Discover how our classes can get you started with game design.

8 Organizations Helping Girls & Women Build Careers in Tech
There’s a gender gap in tech — but it’s getting smaller thanks to organizations like these.
C Functions
C structures, c reference, c exercises.
You can test your C skills with W3Schools' Exercises.
We have gathered a variety of C exercises (with answers) for each C Chapter.
Try to solve an exercise by editing some code, or show the answer to see what you've done wrong.
Count Your Score
You will get 1 point for each correct answer. Your score and total score will always be displayed.
Start C Exercises
Start C Exercises ❯
If you don't know C, we suggest that you read our C Tutorial from scratch.

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Fast & Clean Help with Programming Assignments, Coding & Computer Science Homework
When you need fast programming help, coding assistance, or practical aid with most various types of computer science-related homework - CodeBeach is exactly the kind of service you need. Our nerdy experts are on duty 24/7, ready to provide affordable homework help programming tasks require. We are here to let you crack the code of easier and better studying!
How It Works
Place an order. Fill out the order form as follows:
- Register on our website using a valid email address, Google, or Facebook account, and open the order form. Alternatively, you can do that before
- There, select ‘ Calculations / Problem solving ’ as a type of service and set the required academic level – high school, undergraduate, bachelor, or professional.
- Then, choose ‘ Programming ’ or ‘ Computer science ’ in the ‘ Subject area ’ field and specify the number of problems/assignments you need to be done.
- Set the deadline. Small assignments can be completed as fast as just 24 hours.
- Save 11% on your first order with coupon code "WOWSALE".
- Give as detailed instructions on how you need the assignment done as possible - this is crucial for completing it correctly. You can also attach any materials you deem necessary.
- Finally, choose the expert category – basic, advanced, or TOP – and add paid Extra services if needed (VIP customer service, text messages, explanations).
Process a Payment
- Proceed to paying for your order safely. Select the method to pay the estimated order cost. Available payment methods include Visa, MasterCard, Discover, JCB, and others.
- Before you process the payment, don’t forget to enter a promo code – if you have one – into the respective field and save up to 15% instantly.
- Regardless of the payment method you choose, your data is protected by the Payment Card Industry Data Security Standard and our strict customer info no-sharing policy.
- Every order you place comes with a set of awesome freebies, including up to three free revisions and free email delivery.
- The CodeBeach service works on a pre-paid basis, meaning we can only start working on your order after you pay for it.
Track and Manage Your Order
- Once the order is paid for, our customer managers will select the best-suited expert to work on your assignment based on their expertise.
- Easily get in touch directly with the assigned coder or programmer via the designated messaging system in the Control Panel.
- Send any additional materials needed to correctly complete the order directly to the assigned expert.
- Track the order progress with the help of a special graph interface in the Control Panel or receive SMS updates if you purchase the VIP Extra service.
- Should you have any questions or issues you cannot resolve with the assigned expert, you can at any moment address our customer care agents, and they will readily help you.
Get Your Assignment
- Once the assignment is completed and checked by the QA department, it will become available in the Control Panel – download it with a single click!
- Alternatively, request it sent to the email you have provided upon registration with the CodeBeach service.
- As a result, get a top-notch, original assignment completed by real masters of their craft within the desired timeframe.
- Also, every dollar you spend with CodeBeach can bring you up to 15% of cashback-like reward credits. Thanks to them, you can get better deals on the next orders.
Why Choose the CodeBeach Programming Homework Service
The most important reason why you should choose CodeBeach.com over other websites is the optimal combination of reliability , quality , and experience . The thing is, our service is the sister company of the long-established custom writing website WowEssays.com. This trusted service has been successfully operating on the market for more than 5 years. Now, the company applies its rich expertise to deliver specialized and practical help with programming assignments, coding, and other STEM disciplines.
Great Service
- 100% individual approach
- flexible & pocket-friendly pricing
- free revisions
- 24/7 highly qualified support
Clean Codes
Well-structured, easy-to-read, clean codes that are simple to execute. Each assignment is checked by the QA dept.
Fast & Timely Turnaround
Depending on the assignment’s complexity level, it can be done as quickly as 3 hours or overnight, always on time.
Practicing Professionals
About 90% of our experts are certified programmers and developers employed at IT companies around the world.
Various Delivery Forms
The completed assignment can be delivered as a source code, output screenshots, answers to questions, and other forms.
Rock-Solid Guaranties
- originality
- 100% customer confidentiality
- absolute payment safety
- straightforward money-back guarantee.
A saying about programming goes, “There is an easy way and a hard way. The hard part is finding the easy way.” The Code Beach service has done the hard part and now offers you the easy way to get whatever programming assignment you have done according to the highest standards.
Homework and Assignment Programming Help in Any Language
Having several hundred highly competent coders, developers, and other techies on staff, we can provide online programming homework help in virtually any language:
- Objective-C
- Visual Basic
- MATLAB Assignment
As you can see, we can deal with programming assignments and homework in languages and environments used by both beginners and experienced coders. What’s even more important, we can get the job done following the precepts of Kent Beck, the creator of extreme and agile programming: make it work, make it right, and make it fast!
Custom Programming Assignment Help in Various Areas
As a result of having hundreds of prominent coders on staff who perfectly command various programming languages, Code Beach can provide practical homework help in programming in virtually any software development shell or method, web environment, modeling, data and statistics-related disciplines, including:
Programming
Tech assignment
Calculations
Problems solving
- Programming languages
- Web programming
- Desktop applications development
- Mobile applications development (iOS / Android app development)
- Database design and optimization
- Data science (Data analysis and reports/Data preprocessing and visualization )
- Computer science
- Computer networking and cybersecurity
- Cloud networking and computing
- 3D CAD modeling
- MYOB and others
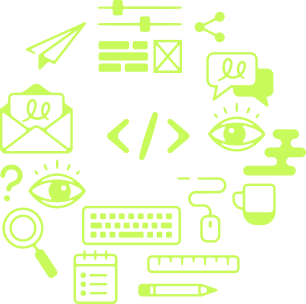
Depending on the assignment area, programming language, and other requirements, your order can be delivered in various forms to best suit the purpose:
- Clean source code;
- Responsive webpage;
- Responsive website of multiple pages;
- Web-based product with several scenarios;
- Solutions to practice problems;
- Answers to theoretical questions;
- Lab report;
- other forms required by assignment instructions.
Each assignment comes accomplished according to the industry’s best practices, compliant with the latest language or environment documentation, and well-commented for you to understand the logic behind the solution.
Original & Clean Code by the Best Programming Assignment Helper
Although plagiarism is more of a problem in academic writing, it can still harm your aspirations in programming. The thing is, while programming plagiarism is not considered something out-of-the-line in real life due to the vast number of free-to-use repositories and libraries, it might cost you dearly if you’re accused of it in an educational institution. To avoid this trouble, all help with programming assignments and homework from the CodeBeach service undergoes a programming originality check (whenever it is possible to) and contains accurate references to every bit of code.
Naturally, much of the credit for high-quality assignments goes to experienced and meticulous coders and developers we have on staff. Before we even let them close to clients’ orders, they must:
- Showcase great skills in coding and programming;
- Prove their credentials, diplomas, certificates, etc.;
- Successfully complete multiple tests on tasks related to their areas of expertise;
- Show good command of English;
- Get trained and exercise in fulfilling orders in accordance with our standards;
- Complete a probation period under close supervision, get assessed, and pass the final test.

As a result, only the best masters of their craft who can devote their full attention to completing your orders get hired as CodeBeach experts. In a way, if a programmer is a machine that turns coffee into code, then we have the best machines in the industry and enough coffee to code virtually anything!
Overcome Any Programming Challenge with Professional Coding Assignment Help
Developing apps, working with data, or writing code are fraught with numerous challenges. Various programming languages, IDEs, and assignment types make them even more troublesome. Most typically, programmers need help with coding assignment because they face the following problems:
- Intricate syntax due to language diversity and complexity of many of them;
- Bugs and errors that affect code execution and maintenance;
- Code readability and comprehensibility;
- Code efficiency, performance, and optimization;
- Compatibility and integration with APIs, libraries, or other components;
- Scaling and maintainability as projects develop and grow;
- Minimizing vulnerability and enhancing security;
- Tight time frames while adhering to strict quality standards;
- Keeping tabs on the latest technological advancements.
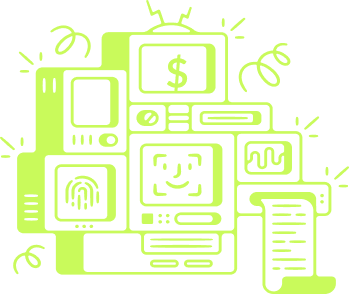
If you realize you cannot cope with some of them alone, hire an expert developer, a pro data scientist, or a coding assignment helper at CodeBeach. Not only can this help you with particular assignments, but it can also be seen as one of the most effective investments in your academic career, maybe even more effective than tuition fees. The thing is, students spend the best years of their lives stressing over assignments that might have nothing to do with real work. Unlike colleges and universities, CodeBeach doesn't tell tales about a prestigious diploma and quickly finding a high-paid job after completing the programs and leaving you with $50 thousand in debt afterward. We just help you do what you must do step by step to let you achieve your academic goals easier and faster.
When to Address Help with Programming Homework or Assignment
Without a doubt, the best way to learn to code is by doing it single-handedly. However, real life is not always as simple as 0 and 1. You must consider that there is no Ctrl-Z in life or in college, so you’d better do everything right from the first try. And that’s when programming homework help from the CodeBeach service comes in really handy!
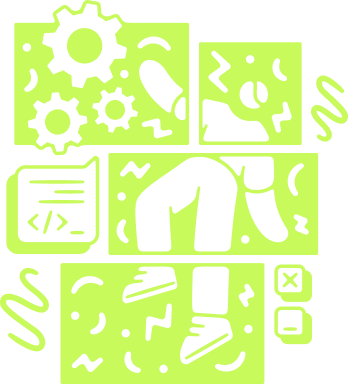
Here are several reasons and circumstances when addressing practical help with programming assignment might be fully reasonable:
- Critical lack of time that you cannot compensate for in any rational way.
- Reluctance to delve into other programming languages you’re not planning to use.
- Using assignments you get as examples to learn from to complete similar tasks single-handedly.
- Lack of understanding of what is required from you to complete the assignment.
- Perhaps, just needing a break and a good sleep and nailing other tasks with renewed vigor.
- Debugging or fighting code decay that may show up with time.
Whatever your reason for addressing Code Beach is, be sure that our experts will help you bring your programming homework up to code!

Help with Coding Homework Tailored to Your Needs
Should you decide to address our service, you can count on full attention from our customer care agents and completely personalized coding homework help from our experts. We preach and practice an individual approach at each stage of your CodeBeach user journey.

- For starters, we love getting clear and detailed instructions from you. So, when placing an order, make sure that you provide more than just its basic parameters like subject area, size, and deadline. Give us specific information on how exactly you want things done and with which software; share thematic guides, notes, or sources; and attach any other materials you deem necessary for an expert to do the job the best way possible.
- Then, you can choose an expert category to fulfill your order. The first thing you should know is that the experts from the Basic category are assigned by default and for free. Alternatively, you can request an Advanced or TOP specialist to complete the task. They have impressive expertise and the best customer feedback but come at an additional cost. Finally, if you've already ordered from us, you can have any of the experts you hired previously complete your assignment.
- Take advantage of our Extras to boost your user experience. For example, get VIP service that prioritizes your order and support requests. Or confine to just Text messages to stay updated on the order's every status change. Also, you can ask for Basic explanations for STEM problems or coding assignments.
- Next, you can contact the assigned expert directly and discuss the order's progress. Furthermore, you can share ideas and send additional documents if something important or interesting comes up after you place an order. And, of course, you get 24/7 support from our agents should you have any questions or issues.
- Before sending the completed order to you, we run it through the Quality Assurance department. Like in virtually any IT company, the team of picky QA analysts meticulously checks an assignment for compliance with the customer's initial requirements and coding's best practices and generally accepted standards.
- Finally, if you still don't like something about the delivered order, we provide free revisions . With them, you can request virtually any amendments that don't contradict the initial order requirements. We will readily adjust the assignment until you're completely satisfied.
Our assignment service comes with a few benefits
Customized approach
Tell us what type of assignment you need, and we’ll find you a personal expert who is qualified to complete the task
Free revisions
You can ask your expert to edit the assignment after it’s been completed so that it fully meets your requirements
Money-back guarantee
If something goes wrong with your order, we will provide a full or partial refund based on each individual case
Data Security and Privacy
We do not share or disclose your personal data to third parties when providing help with programming homework.
Code Beach Programming Homework Help Reviews
web-development / javascript
Took me a while to find a service that specializes in real programming help online with Java, not just tips or examples. Most def recommended.
web-development / python
Real geeks right here! I was completely lost with my fourth Python task, getting urgent assignment programming help was the only solution. Glad that it worked 100%.
database & data processing / sql
Great help with my SQL assignment – on the subject and on time, with detailed explanations. Recommended for data-related homework.
web-development / kotlin
I know there are dozens and dozens of Android development tutorials and resources, but they are no help when you need a Kotlin assignment done overnight. On the other hand, CodeBeach is.
Cranky Frank
3d modeling service
Great 3D modeling service, timely delivery, proactive support agents, and the result as expected. Prices aren’t that high if you order in advance.
whiteRabbit
web-development / php
The most satisfactory result, even considering that PHP is a quite simple language itself. The website can certainly be used for uncomplicated tasks and homework.
swift assignment / ios
Swift service with the Swift assignment and a good helping hand with coding for iOS. Quite affordable, too. Just be as detailed with your instructions as you can to avoid revisions later.
It’s as simple as the ‘if-else’ condition: if you cannot do an assignment yourself, then you’ll fail; else: hire someone who can do it for you right and fast.
if_then_else
Is getting computer programming homework legal?
The good news is that getting online programming help is as legal as using a library or addressing a tutor – there are no regulations that prohibit that. Add here that CodeBeach, as well as its sister company WowEssays, is a completely legit company, with the latter being almost 10 years in the custom writing industry. The shady part is that you are not supposed to submit papers and assignments you get from us as your own unchanged. While we ensure assignments’ originality and customers’ full confidentiality, we also don’t condone plagiarism and cheating.
How much does programming assignments help cost?
As Code Beach has a flexible pricing system, there’s no fixed cost we can present you here. The final price depends on many factors, including the complexity and the size of the assignment, the expert level you need to get it done, the assignment’s type, the required language, and of course, the deadline. Use the order form to get the estimated cost or contact our customer managers, who will perform the estimation for you.
Do you have discounts for homework help programming orders?
Yes, CodeBeaches offers several saving opportunities for its customers. For starters, all new clients who address our programming help are by default entitled to a special welcome offer. It may include an instant discount, a discount combined with loyalty reward credits, or a special feature provided for free (for example, VIP service). Then, there’s the Loyalty Program that lets you accumulate reward credits for your orders and then use them to pay for the next orders. Finally, if you have a large order, we suggest you get in touch with our customer managers and inquire about a one-time discount and/or the progressive delivery possibility
Can I contact the assigned expert while they’re working on the order?
Yes, the possibility to contact an expert assigned to your order is one of the features CodeBeach has implemented to ensure a truly individual help with programming homework. Once we find the best-suited expert to fulfill your order and you pay for it, you will be able to contact your coder or developer directly via the designated messenger system in your personal cabinet.
Can your experts do real-time online assignments and tests instead of me?
That’s a strong no. We help but do not do something instead of you, especially real-time online assignments and tests. This is a severe violation of the academic integrity principles. We can deal with almost anything you can send us via the order form but don’t expect our expert to log in and pass an online test instead of you.
How do you ensure customers’ confidentiality and payment safety?
Customers’ confidentiality and payment safety are among the top-4 of CodeBeach’s priorities, along with the delivered product quality and overall user satisfaction. To this end, we’ve secured our website through SSL encryption and implemented a strict privacy policy that excludes sharing whatever client data we have with third parties. The compliance of our website with the globally recognized Payment Card Industry Data Security Standard requirements comes as a topping. It ensures that all your payment data will remain absolutely secure. Thus, using the CodeBeach service is completely and utterly safe.
Deadline is running out?
Don't miss out and get 11% off your first order with special promo code WOWSALE
No, thank you! I'm ready to skip my deadline.
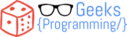
Need Programming Homework Help?
Simple, No-frills Programming assignment help and tutoring Service, Just submit your problem / Homework -> Get the help.
Best Programming help with modest pricing. No fumbling around the expert profiles yourself, No complex forms or hourly rates. Option to solve your programming assignment 1:1 online with an expert—because growth happens when we collaborate.

Average Rating
Satisfied Students
Years in Service
Programming Languages
#1 Priority
Privacy & Confidentiality
HANDOVER YOUR ASSIGNMENT
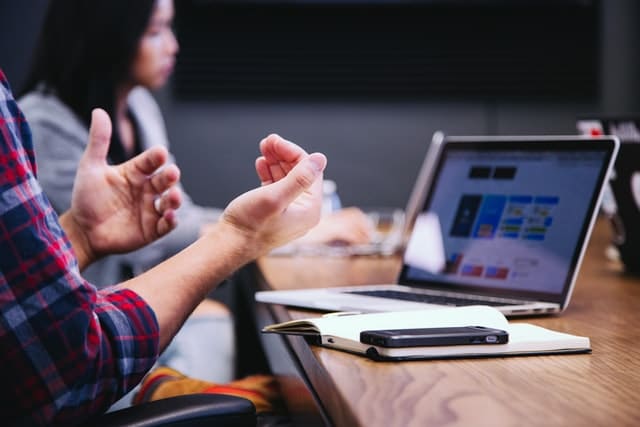
Let Us Worry
Having Panic Attacks and Worrying About Approaching Deadlines Will not Help you, but we at GeeksProgramming Definitely can help with programming homework & assignments.
TRACK PROGRESS
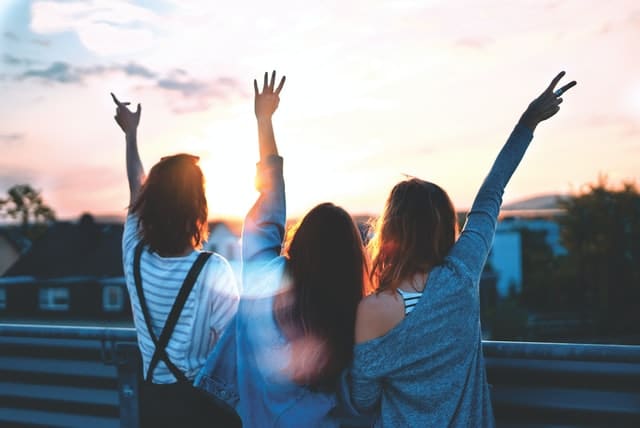
Relax and Enjoy
Go Hangout with friends or watch some TV while we are working on developing and coding your programming homework. Get the best Programming assignment help service.
SUBMIT YOUR ASSIGNMENT
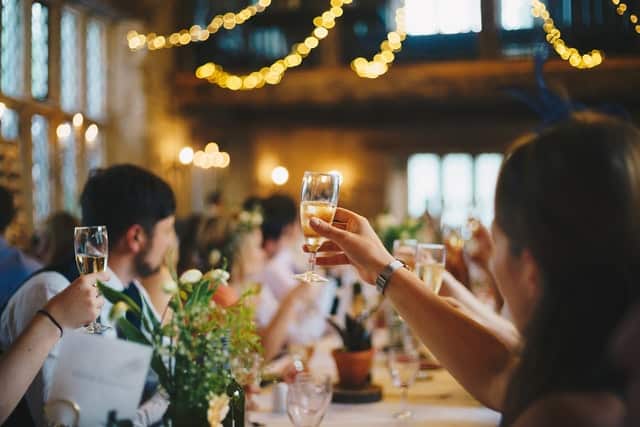
Get Best Grades
We are expert Programming Homework Help and Programming assignment help services provider, and no chance you will miss the Best Grades in the class on our work.
Why, How and What we do?
What we do.
It’s simple- We render the finest Software Development & Programming Assignment Help to all our clients. Our teams can perform functional coding operations and follow ethical programming practices, including:
- Agile Methodology
- SOLID design principles in OOP
- XP (Extreme Programming) or YAGNI (You Ain’t Gonna Neet It)
- DIE (Duplication is Evil), DRY & KISS principles
We are best at what we do. Give us a chance to show you that. Contact our executives and get your work done!
Why GeeksProgramming?
Geeks Programming is your one-stop platform to get Programming Homework Help at the most affordable prices. We provide this service to help out students, freelancers, entrepreneurs, and businesses so that they can get help when they are stuck at something.
With a team of exceptionally qualified and well-trained professionals, we ensure that all your assignment related problems are solved. Do you need some computer programming help? No worries. We have got your back. Our teams work with sheer commitment and drive to get your assignment done on time and take some load off your shoulders.
The sole reason we have entered this field is that we know how difficult it is to get last-minute projects or programming assignments done. We wish to make the assignment completion process easier and better for you. We will be making money by assisting you rightfully.
How We deliver perfect solution every time?
When people ask us how we provide best in class programming homework help, our answer is simple 3P Passionately, Perfectly and Professionally!
With love for programming in our veins isn’t that obvious? We have several professionally trained and experienced programmers in our team. All our programmers have a unique skill set and are devoted to their profession. They ensure that they put 100% of their skills in completing the assigned project work.
You just have to contact us and submit your coding assignment guidelines. We start our working to finish your assignments and give them a prolific touch.
When? Deadlines!
When can we help you out? Always! Our Programming Help services are available for you 24*7!
Feel free to contact us using contact form, facebook chat or give us a call. We will always revert the earliest possible.
If you are worried about missing your deadline, then sweep this concern away. You are free to set the due dates or deadlines with us. We will follow it properly and deliver it on time
How much will it cost?
Our pricing policies are much similar to other providers yet different. We follow a fully customized pricing plan for every project we attend to. The price of every Programming Homework Help is different than others.
Our pricing is based on what your requirements are and how much efforts will the project require. Prices start at $50 for simple assignments in generic programming languages. It can go above thousands depending on the assignment – Blockchain, AI, ML, and various other professional topics and subjects.
We know that it is hard for students to pay a lump sum amount for getting coding homework help. This is why we keep our prices genuine and support partial payments so that they can get the timely help they deserve.
Inclusions and Add-ons of Our Coding Homework Help Service
Plus assurance of 10 years of GeeksProgramming
Included in Quoted Price
- Expert Developers from top-tier Universities around the World
- Unique and Plagiarism Free Solution
- Step By Step Guide on How to run the code
- Unlimited Revisions Free of Charge
- Well Commended Code and Documentation
- Anonymity and Confidentiality of your Identity and Data
- Simple and Full Refund Policy
Affordable Add-ons
- Guided Live Assignment Help
- 1:1 Live Session with the Programmer to help you understand the Assignment & Solution
- Deployment of the Solution on your Server
- Certified Plagiarism Report
- Assignment based viva voce & Interview Prepration with the expert
- Online Exam Prepration & Tutoring
- Course based & On Demand Tutoring
For a Decade, We've Passionately guided and uplifted students worldwide.

- Nearly a decade in the Programming help services! – We’ve been smashing programming assignments out of the park, gaining a solid rep for our tutoring services. Our secret? Keeping disputes low and satisfaction high.
- We understand you, All you’ve got to do is shoot us your programming assignment, and then sit back and relax while we handle the rest.
- Our programming experts are pros at Tutoring, who can whip up code that’ll look like it came straight from a student’s keyboard. Easy peasy to understand!
- We know that as a student, every penny counts. That’s why our prices are super affordable. No need to break the bank for an assignment or homework.
- We’re all about being punctual. We know every second counts for you, so missing a deadline? Not on our watch! And if we ever goof up, we’ve got a ‘no excuses’ policy where we refund 100% of your money. So, if you’re choosing us for your programming help, rest assured, your solution will arrive right on time.
- From novice to ninja-level programming projects, our skilled experts have got you covered. Just toss us your requirements and then sit back and watch us do our magic.
Why should you get Programming help from us?
Here is a brief overview of the features you can expect from our service.
Trouble free Assistance
On chat or email, our coding assignment help will feel like getting your work done by a close friend. Need help with programming? or running the code.
Skilled Staff
We have the Industry’s best programmers and developers. Who work diligently in making a solution that fits your specifications perfectly.
Hi-Tech Help Provider
From C++, Java, Python to ML, etc. Our experts will make your submission the highlight of the day. Using programming help will get you top grades!
Available 24x7
Regardless of Day and Night, feel free to contact us. We will solve all your queries and ensure that your coding assignment is completed and delivered on time.
On Time Every Time
Our Mantra- “Pre-Deliver & Over-Deliver”. We abide by the fixed deadline. It makes easier for us to make any revisions that you need at the last moment.
Reliable Support
A customer support team that can help with programming homework anytime. And they are just a text or email away. Assured- You will get an instant reply.
Zero Plagiarism Promise
We create tailor-made solutions for all your Programming Homework Help needs. Starting from scratch, we follow requirements strictly to maintain the content’s originality and uniqueness.
Confidentiality Guaranteed
Your confidentiality is our utmost priority. We keep your data secure, meaning your information stays encrypted and safe.
Meet GeeksProgramming Teams
These are the teams of real Heros who work behind the curtains to deliver the best Programming Help on the web

Team of Project Managers
Your initial conversations for Coding Homework Help would be with our Project Managers. Our Project Managers have extensive knowledge of programming languages and their applications, so they know what’s best for you. They will hear you out, and after keeping in mind all your requirements and specifications, they will assign the task to the best developer or programmer.
Team of Subject Experts
The expert team is our biggest strength. Having experience in serving clients from different places in the world, they are more than capable of completing your assignments. After being allotted assignment tasks by the Project Managers, they get to work and complete the work timely. Also, we keep your personal information confidential from our developers to maintain strict privacy.

Team of Customer Support
If you have questions, doubts, or complaints about our Programming Homework Help services, you can contact our assistive Customer Services team. They are well-equipped and trained to give answers to all your queries. They are available at your disposal throughout the day so that none of your doubts are left unresolved
OUR SeRVICES
It's like Concierge Service for Programming help.
Our Programming Aid is customised as per your requirements. That’s why we don’t have fix set of pricing lists. We provide a custom price quote for every enquiry and not only it is flexible as per your requirements but affordable as well. Prices for basic assignments start only at $50.
Here at GeeksProgramming, every time you enquire about an Assignment, our Project managers go through them and assign an Expert based on the requirements. So you don’t have to fumble through the options and choices of experts or freelancers. Below is just a list of categories of Coding help services; however, we cover everything related to STEM (Science, technology, Engineering and Mathematics).
Whenever you feel that you have got a lot of projects, assignments, and studies to do, give Geeks Programming a call! If your programming help is very complex and difficult, don’t hesitate in asking for help. We render our assistance for Java, C++, PHP, Python, and other programming homework.
We will not only complete your assignment but also help you clear our queries while we are working on your assignment. Our adept team will add a refined touch to your project work making it even more impressive. You will receive straight A’s after submitting a project made by our expert developers.
So, the next time you are wondering how will you complete so much work? Have no worries. Leave it to us. Geeks Programming is available 24*7 for help.
Find more about How can I hire someone to do my programming homework?
No matter whether you have an approaching deadline or are burdened with work making it impossible for you to complete your work. You can always trust us to get you the finest Programming Homework Help! If you have tight schedules and need help with your assignment urgently , then Geeks Programming is at your service.
After hiring us to complete your assignment, you won’t have to worry about your deadlines at all. We will make sure that your project work is delivered to you well before the final due date. Relax and give us a call, we will complete your homework and clear your doubts in a jiffy!
Geeks Programming also caters to all your Java Assignment help needs. We have successfully rendered this service to our clients from different parts of the world. Ranging from solving complex doubts about Java to completing your homework or project work for you, we do it all! Our programming homework help will not only get your name among the class toppers but will also give you a better insight into Java and its concepts.
Our goal is to get you an enhanced understanding of Java, writing syntax, various coding patterns, encapsulation, and abstraction. Our team has a lot of Java programming experts; they will assist you throughout the assignment completion.
Read How to get someone to help with java homework?
Another mind-boggling subject for not only students but also working professionals and freelancers is C++. We have extended a helping hand to all those who are going through the same problems. Geeks Programming has a solution for your entire C++ related doubts and assignment completion issues.
If you already have multiple assignments to complete, then Geeks Programming is here to rescue you from your C++ project work. No matter if you are a C++ expert or not, we will help out always. We will charge a nominal amount from you to solve your most complex C++ assignments and projects. Our services have helped various students in receiving good marks in their academics
Do you frequently think that Python Assignments are very difficult? Or are you having a project submission date soon? Don’t lose your sleep for this. Relieve your stress and hand over your assignment tension to us. Regardless of the assignment complexity and difficulty level, our specialist programmers timely complete your assignment.
Our developer teams are ready and waiting for you to give us the baton while we finish the race for you! You can rest assured that signing up for our services will get you good grades in your classes. Our around the clock support makes it easier for students from different parts of the world to avail our python programming help.
If you feel that you won’t be able to score well or your assignment will look very unprofessional, then we are here for you. We understand that not all students like studying programming languages or are not equally skilled in the field. This is why we provide Database and SQL Assignment Help.
Our programmers and developers are informed about your assignment requirements, after which they start their work on the project. We double-check the assignments for any mistake or change, only then we send the final work to you for your approval. With the best value for your money, we charge our clients inexpensively for all our work.
We are also experts in providing help to our clients for completing their PHP assignments. Our developers and programmers have extensive knowledge of PHP programming; they can get your PHP assignment work done within the preset deadline. They not only perform research but also apply their skills to give you the PHP assignment of your dreams.
We recheck every assignment we do before delivering it to our clients and leave no room for error. Your unique and fully customized assignment will make you stand out
Android assignments are creating another area of concern for all the students. Most of the time either they submit their assignments to get average grades or miss their deadlines. At Geeks Programming, our help experts go through your guidelines and create an exceptional project before the final submission.
To give you a better knowledge of what we do, we also answer your queries throughout the assignment making phase. With their expansive knowledge of the field and years of experience, we can assure you that you will get the best Android assignment help without any plagiarism.
Find How to get CS Homework help online?
Hire Prolog Programmers at Affordable prices
GeeksProgramming, have your back when it comes to Stats assignments help.
Recent Blog Posts
Ultimate guide to deciding architectural design in software engineering, master java networking: sockets, servers, urls, and rest apis, spring batch: the ultimate guide, how is the quality of code / work we do check example below.
We are committed to providing top-notch Coding help services that adhere to the highest standards of coding and clean code practices. Our features include:
Coding Standards: Consistency & Readability, Write code that is easier to read and Understand. Consistent naming convention, indentation style, and file structure . Proper documentation , robust error handling , and thorough testing are essential for maintaining code quality and reliability.
Our clean code practices at GeeksProgramming prioritize simplicity , clarity , and organization , focusing on concise functions, descriptive naming, logical structure, c ontinuous refactoring , and regular code reviews to ensure maintainable and efficient code.
However, if you want your code to have a beginner or amateur vibe , we’re happy to tweak our standard practices just for you! 😉😉
How to get Online Programming Homework Help?
The process is easy, get in touch & tell us exactly what you need.
There are various options available to reach us. Choose the best that suits you, such as E-mail or chat. Submit your programming Assignment, Homework with all the Instructions and Necessary Details.
Pay token Amount & Track Progress
You will receive a competitive price quote tailored to your specific requirements. By paying 50% of the total upfront, you can initiate the project. Relax and monitor the progress while enjoying your cup of coffee.
Get Complete Assignment & Ask for Revisions
Once your assignment is complete, you will be notified. By paying the remaining amount, you will receive the full solution. You can review it and request free revisions or modifications if needed.
Frequently Asked Questions
We have tried to answer the most common queries students may have. If you have more questions or concerns beyond those listed below, you can reach out to us and ask.
What is the Order Process?
Are the solutions/assignments provided plagiarism free, how long will it take to complete a task, is geeksprogramming legitimate, what is the best website for getting online programming assignment help.
When it comes to sorting out those tricky programming assignments, GeeksProgramming is a real lifesaver! It’s made a name for itself with its high spots in search rankings, which pretty much says a lot about how folks prefer it over other platforms. It’s like go-to spot for programming help. Its super easy and straightforward get the help you need. Plus, the prices are student-friendly, and the experts there really know their stuff. So, when the coding gets tough, GeeksProgramming is definitely a solid choice to consider!
Do you offer help with advanced topics like AI, Blockchain, or Machine Learning?
Yes, we offer assistance with advanced topics like AI, Blockchain, and Machine Learning. Whether you're conducting research on neural networks, stuck on a complex algorithm, or need to develop a project from scratch, just let us know your requirements. Our team of expert programmers will handle it with precision. Additionally, we provide 1:1 support to help you understand complex projects and enhance your knowledge, ensuring you're fully confident in the material.
How do I track the progress of my programming assignment?
After placing an order with us, we understand how important it is to stay updated. That’s why we provide progress updates every 12 hours, ensuring you can track the project in real time. This gives you peace of mind and the opportunity to request any changes along the way if needed. Our goal is to keep you fully informed and involved throughout the process, making sure everything meets your expectations.
Can I interact directly with the programmer working on my programming homework?
Yes, you have full access to communicate with the programmer at any time. We are always happy to arrange a meeting, even at the start of the project, to clarify requirements and ensure the tutor is a good fit for your task. After placing the order, you're welcome to ask any questions, and the expert will respond directly to address all your queries. Our priority is to keep the communication open and ensure you’re confident throughout the process.
Where can I get help for programming?
How much does your service cost, which payments forms are acceptable, what is the refund process and criterion, is your service confidential and secure.
Yes. We take the utmost care of our client’s privacy and do not share your personal information with anyone. All the communications and data stored are always highly encrypted.
Do you offer tutoring for students to help them understand their assignments?
Yes, we offer personalized tutoring for any specific programming subject. Whether you're stuck on a challenging topic or need guidance with a programming assignment, our 1:1 tutoring sessions allow you to interact directly with our experts. If you've hired us for programming homework and would like to understand the assignment in detail, simply let us know. Our tutor will walk you through the entire project via screen-sharing, ensuring you grasp every aspect of the work.
What happens if my assignment requires specific software or environment setup?
If you require us to use specific software or set up a particular environment for your project, just let us know, and our expert will follow your instructions. Even if you forget to mention it initially, you can inform us at any point, and we will adjust accordingly. If you face any issues running the code on your system, our expert will connect with you to ensure everything is set up correctly on your PC, making sure the code works seamlessly without any problems.
Can I request revisions after the assignment is completed?
Yes, you can request unlimited revisions until you are fully satisfied. Our programming assignment experts are always available to make any necessary changes to the code if it doesn’t fully meet your requirements. While we strive for perfection in every task, occasional errors can occur since all our code is handcrafted by our experts. If any adjustments are needed due to missing or unclear requirements, we will make them without any extra charge, ensuring the final result aligns with your expectations.
GeeksProgramming Reviews
What do our clients say, looking for a first-class programming help, get a free quote, want desired grades – sit back & relax. our experts will take care of everything..
Get an expert's assistance with your coding homework
Get fast and high-quality solutions for your computer science tasks. We can cover any complexity, discipline, or academic level.
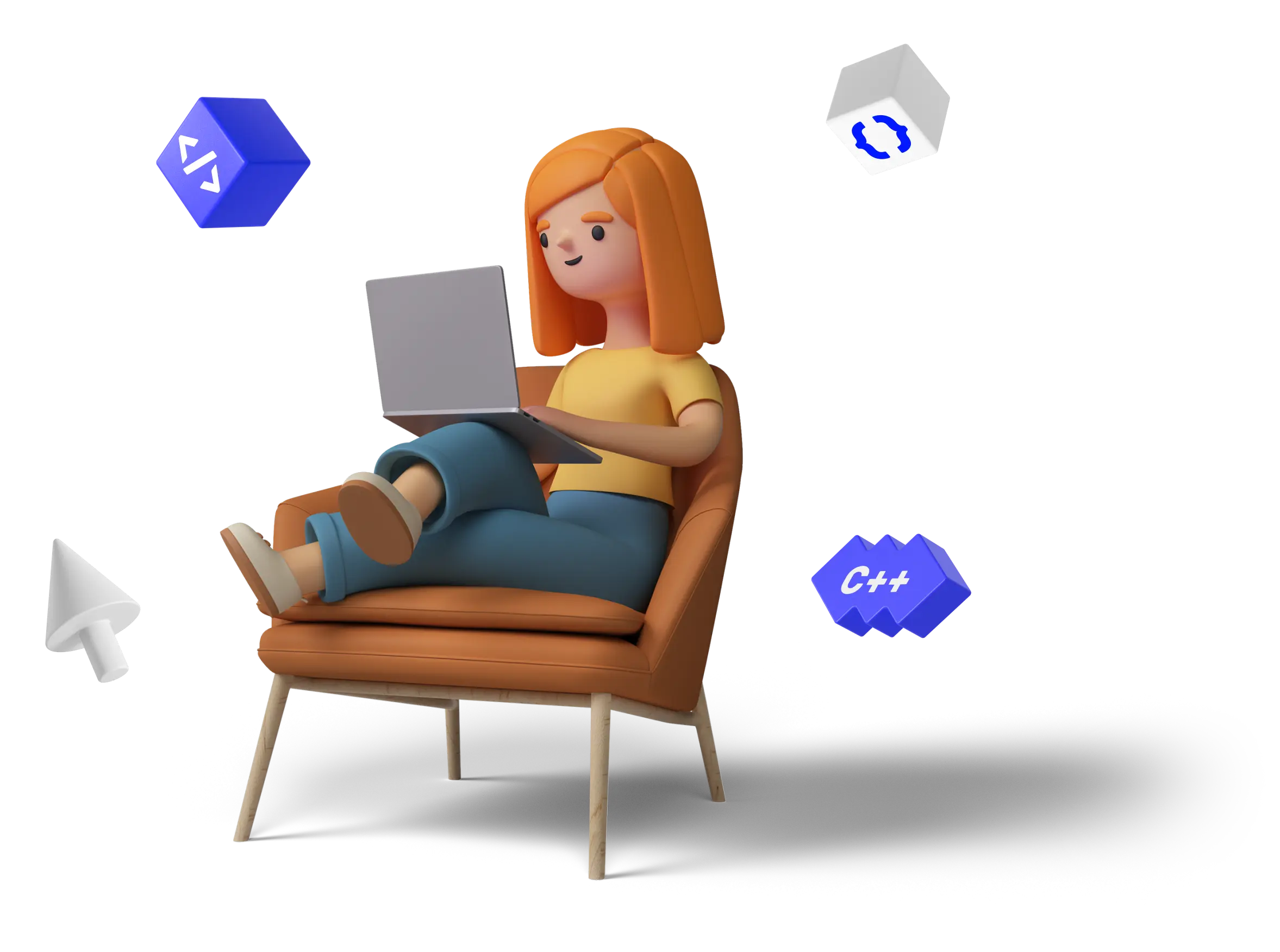
Get solutions in 30+ coding disciplines and more:
You can reduce the price of your assignment by extending your deadline.
We check if our solutions meet your requirements and fix our materials for free.
We can issue a partial or full refund if you’re not quite satisfied with our solution.
We complete your task according to your requirements.
We can deal with the simplest programming tasks in a few hours!
Find out the price of your programming assignment
You can reveal the price of your coding task before the placing an order.
How our service works
Follow these four steps to get your programming assignment done.
- Place an order with all the requirements we have to follow.
- Pay for your order safely in just a few clicks.
- Track the progress of your task on your personal order page.
- Get your solution and check if it needs any free fixes.
Some numbers about our work
We’re proud of each percent of our customer satisfaction rate and other quantitative measures of our work.
orders delivered on time
years helping students
quality score given by customers
programming experts available now
Our team members and what makes them special
They complete tasks and move your programming assignment forward. Only 9% of all candidates can become an expert on our team.
Experienced supervisors watch over the quality of your solution. They test and instruct our coding experts to ensure the highest quality of completed materials.
These people work around the clock to make you comfortable with our service. You can ask anything and get direct answers anytime.
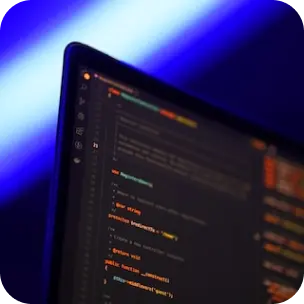
What our customers say about us
Check out the reviews before placing an order to know that our experts can deal with your programming assignment.
Your solution may look like this
Check out the style, code, and creative approach in the samples created by our coding specialists.
Frequently asked questions
1. what should i prepare before placing an order, 2. how can i know your service is legit, 3. can i get a discount on my order, 4. can i ask for urgent help with my assignment.
- All Services
- Python Programming Help
- Java Programming Help
- JS Programming Help
- C Programming Help
- C# Programming Help
- C++ Programming Help
- Matlab Programming Help
- Php Programming Help
Expert programming assignment help service
There is no need for you to worry about your assignment in programming, especially when you can ask for professional programming assignment help online. There are several programming assignment help services today that can provide you with assistance in completing your homework on time. Since there are several sites that you can turn to, it is important that you look for a company that can provide you with high-quality results, which is why we are here to help you out. We know how difficult it is to write code and make sure that it is free from errors. That is why we hired certified programmers to join our team to ensure that your assignment works just fine.
Our team can handle different languages. For example, we provide:
Java programming assignment help
Good old Java is one of the most widely used programming languages, but that doesn't mean that all of its areas are well-explored and easy to cope with. Sometimes it's just impossible to memorize all the classes and figure out how to incorporate them. We are here to do just that.
C++ programming assignment help
Struggling to put the code together and can't concentrate on the big picture? Let us help you out! Provide us with all your ideas and coding drafts, and together we'll be able to create a perfectly functional code!
C programming assignment help
How about all of those functions – confusing, isn't it? You can easily get lost in all the numbers and characters and simply not see what stops your code from working. Our team will be able to detect the bug and fix it in no time.
Professional programming homework help
It doesn't matter what programming assignment you need to do - whether it is in C++, Java, Perl, Adobe Flash, or Visual Basic, among others - we are confident that our programmers can complete your homework on time. Our programming homework help service team has several professional programmers who have the skills as well as experience in handling various programming homework where you can even discuss details of your order so you can get the best result.
Programming assignment done fast
It is understandable that you'll feel frustrated with your programming homework, especially when you have other subjects to consider. Instead of spending most of your time writing code and debugging it, having expert programmers handle your work is worth it, especially when you get to see the results. And since you'll be working with our programming homework help service for your programming expert assignment, relying on the skills of highly-qualified programmers will certainly go a long way.
Get Professional Help With Any Programming Assignment
How it works, what you get, work samples, 2,300+ customers love our service..

An experienced coder at programmingassignment.net can do your programming assignment fast and up to the highest standard. From Java to C++, we have experts in almost all programming languages and handle projects of any complexity.
The total price greatly depends on what exactly needs to be done and the preferred turnaround time. Our prices are one of the most affordable online and start at just $45 per assignment. Please share detailed assignment instructions with us, and we’ll provide you with a price quote.
If you cannot solve a programming assignment, the best option will be to consult a subject-matter expert like the ones we have here at programmingassignment.net. Our experts in Python, C++, SQL, Java, and other languages can not only help you handle any programming assignment efficiently but also provide detailed explanations of all the methods and functions and offer a step-by-step guide on how to run the code.
You can find professional programming assignments help with guaranteed quality at programmingassignment.net. Our dedicated team is available to assist you 24/7, and our fastest turnaround is just 12 hours.
It all depends on the assignment’s complexity. On average, small programming assignments take 1-2 hours to complete, whereas more complex ones require 2-3 working days.
When our manager receives detailed assignment requirements, they find an appropriate programming expert for you and email you a price quote along with a payment link. As soon as you pay for programming homework help , the expert gets down to work.
Please share your assignment requirements with us. Our experts will carefully examine them and help you select the most appropriate programming language and environment.
Our minimum turnaround is just 12 hours, so rest assured we’ll be able to assist you with the task.
Absolutely! Protecting customer privacy is our top priority, and all of our employees are bound by the NDA and legal agreements. Opting for our homework help programming , you can be sure that no information about your order will be disclosed to a third party.
Yes, that is possible. According to our Terms of Service, the completed task can be revised for free within two weeks from the moment you receive coding assignment help at our service.
Depending on the customer’s needs, an internal system called iBPS assists us in selecting the best programmers for the task and makes it simpler to assess their performance. In addition to customer feedback, the evaluation also considers 11 additional parameters.
- UML Diagrams Assignment Help
- Lisp Assignment Help
- PHP Assignment Help
- OpenGL Assignment Help
- OpenCV Assignment Help
- Assembly Language Assignment Help
- Arduino Assignment Help
- Computer Networking Assignment Help
- Programming Homework Help
- Programming Assignment Help
- Coding Assignment Help
- Advanced Web Technologies Assignment Help
- Computer Security Assignment Help
Unparalleled Programming Assignment Help Provided by Tech-Savvy Professionals
Our unwavering commitment lies in assisting students in crafting clean, comprehensible, and effortlessly executable code. With over a decade of trusted expertise in providing programming assignment help to clients worldwide, we stand as a beacon of reliability. Our dedicated team of tech-savvy professionals tirelessly codes throughout the day and dedicates their nights to meticulous debugging, ensuring the swift delivery of flawless programming projects. Trust us to be your coding ally on the journey to success.
Your Go-to Platform for Online Help with Coding Assignments on a Wide Range of Topics
CodingAssignmentHelp.com stands out in writing coding assignment due to our team's in-depth knowledge, experience, and dedication to delivering high-quality solutions that meet the unique demands of each subject area. Our programming experts are well-versed in the intricacies of various coding topics, allowing us to provide unmatched assistance to students seeking help in these domains and more:
Subject/Topic | Description |
---|---|
Web Development | Students receive support in web development projects, including HTML, CSS, JavaScript, and web framework assignments. |
Data Science | We help students with data science tasks, from data analysis to machine learning, empowering them in this cutting-edge field. |
Database Management | Our expertise in database management helps students design, query, and optimize databases for their projects. |
Mobile App Development | We assist in mobile app development tasks, guiding students through iOS, Android, or cross-platform app development. |
Algorithms & Data Structures | We provide solutions and explanations for algorithmic challenges, enhancing students' problem-solving skills. |
Artificial Intelligence | Our AI expertise supports students in AI-related projects, including natural language processing and computer vision. |
Software Engineering | We aid students in software engineering projects, ensuring they follow best practices and produce high-quality software. |
Embedded Systems | We offer specialized support for embedded systems, helping students develop efficient, real-time applications in constrained environments. |
Desktop App Development | Our guidance covers desktop application development using Java and C++, enabling students to create robust and user-friendly software. |
Assembly Language | We assist students in mastering assembly language programming, facilitating their understanding of low-level computer architecture and coding. |
Established & Renowned Provider of Affordable Online Programming Assignment Help
At CodingAssignmentHelp.com, we have earned our reputation as a renowned provider of affordable online programming assignment help by placing students at the forefront of our mission. Recognizing the diverse financial backgrounds of our clients, we have meticulously customized our rates to ensure that quality coding assistance remains accessible to all. Our commitment to affordability is rooted in the belief that every student, regardless of their budget, deserves the opportunity to excel in their programming assignments and programming coursework. Our pricing structure is designed to accommodate various assignment complexities and deadlines, providing flexibility to students while maintaining the high standards we uphold. To illustrate our commitment to affordability, here's a table outlining our pricing ranges:
Assignment Complexity | Price Range (USD) |
---|---|
Basic assignments | $45 - $80 |
Intermediate projects | $50 - $150 |
Complex Coding assignments & Projects | $150 - $500 |
Urgent and advanced tasks | Customized quotes |
Hire Us to Do Your Programming Assignment & Enjoy Jaw-Dropping Discounts
At CodingAssignmentHelp.com, we understand the importance of supporting students not only through high-quality programming assignments but also through financial assistance. To show our appreciation for their trust and loyalty, we offer exclusive discounts and special offers to students who entrust us with their coding assignments. Our commitment to providing exclusive discounts and offers is rooted in our dedication to supporting students' educational journeys while making our services affordable. These benefits not only make our service accessible but also underscore our mission to be a trusted partner in their academic success.
- Second Order Discount: After their initial experience with us, students who return for a second assignment enjoy a special discount as a token of our gratitude for their continued trust.
- Repeat Customer Benefits: For students who use our services regularly, we offer loyalty rewards and ongoing discounts, making it even more cost-effective to work with us over time.
- Bulk Order Discount: We provide discounts on bulk orders, ensuring that as students' academic workload increases, our prices remain budget-friendly.
- Referral Rewards: Our referral program allows students to earn discounts by referring friends or peers to our services, expanding the benefits they receive from working with us.
- Seasonal Promotions: Throughout the year, we run seasonal promotions and offers, making our services even more accessible during holidays, exam seasons, or special occasions.
- Customized Offers: In some cases, we can create personalized offers to accommodate the unique needs and financial situations of individual students.
Popular Programming Languages Covered by Our Coding Assignment Help Service
CodingAssignmentHelp.com's qualifications in various programming languages stem from our team's extensive hands-on experience, deep-rooted passion for coding, and a track record of successfully delivering high-quality solutions. Our team members are not just knowledgeable; they are practitioners who have spent years honing their skills in real-world projects, which makes them adept at addressing the nuanced challenges that often arise in coding assignments. Furthermore, our programming tutors stay up-to-date with the latest developments in these languages, ensuring that they can tackle assignments that encompass both foundational concepts and cutting-edge features.
- Java: Extensive Java development experience, providing expert guidance and solutions for assignments.
- Python: Deep proficiency in Python, including libraries and frameworks, ensuring efficient, error-free code.
- C++: Excellence in C++ programming, enhancing students' coding skills and understanding of C++ concepts.
- JavaScript: Expertise in JavaScript and web development, enabling dynamic and interactive web application creation.
- PHP: Assistance in PHP programming, guiding robust web application and website development.
- Ruby: Focus on Ruby programming, offering comprehensive support for assignments and projects.
- Swift (iOS Development): Proficiency in Swift for iOS app development, ensuring mastery in Apple device app creation.
- Kotlin (Android Development): Support for Kotlin-based Android app development, creating user-friendly mobile applications.
Standout Features that Make Our Website Programming Students’ Favorite
At CodingAssignmentHelp.com, we stand out from the crowd with a distinctive set of features that ensure top-notch programming assignment assistance. These standout features collectively embody our dedication to providing exceptional programming assignment help. We take pride in assisting students with their programming challenges while upholding the highest standards of quality, professionalism, and support. Our commitment to excellence is reflected in the following key features:
Our programming assignments are characterized by clean, well-structured, readable, maintainable, and efficient code. We prioritize code quality to help students understand and learn from the solutions we provide.
We adhere to industry standards and best practices when writing code. This ensures that students receive assignments that not only meet academic requirements but also reflect real-world programming practices.
With over a decade of experience, we bring a wealth of knowledge and expertise to the table. Our rich history of helping students write programming assignments has honed our skills and understanding of the subject matter.
Our team comprises 90+ nerdy programmers who specialize in various topics. These dedicated experts ensure that every assignment is handled by someone with in-depth knowledge of the subject matter, resulting in high-quality solutions.
We provide well-commented and plagiarism-free codes, giving students valuable insights into the logic and reasoning behind the solutions. This helps them not only submit assignments but also improve their programming skills.
We prioritize our students' needs by offering 100% confidentiality, affordable pricing, and round-the-clock customer support. Your privacy is our utmost concern, and our pricing structure is designed to accommodate students from diverse financial backgrounds. Our 24x7 support ensures that help is always at hand when you need it.
Get Your Urgent Coding Assignment Completed in 3 Simple Steps
We understand that your time is precious, and your primary focus should be on getting the coding assignment assistance you need, without the hassle of account creation or registration. We prioritize your convenience and aim to streamline the process, allowing you to access professional assistance swiftly and effectively. By eliminating the need for account creation or registration, we've removed unnecessary steps, making it easier for you to submit your assignment and receive expert guidance. This user-friendly approach ensures that you can quickly navigate our platform, get a quote, and confirm your order without any additional complexities. Availing of our coding assignment help service is hassle-free and doesn't require any account creation or registration. Here are the simple steps to get the assistance you need in just three easy steps:
- Submit Your Assignment Details: Visit our website and navigate to the "Submit Assignment" section. There, you can provide the details of your coding assignment. Include information such as the programming language, specific requirements, deadline, and any supporting documents.
- Get a Quote: Once you've submitted your assignment details, our team will review your requirements. You will receive a personalized quote for the coding assignment assistance. This quote will be based on the complexity of the task and the urgency of your deadline.
- Confirm and Receive Your Solution: After reviewing the quote and ensuring it fits your budget, you can confirm your order and make the payment using our secure payment options. Once payment is confirmed, our expert programmers will begin working on your assignment. You will receive the completed solution within the specified deadline, ready for submission.
Super-friendly Customer Support Team at Your Service 24x7
Our commitment to providing exceptional coding assignment help extends to our customer support team, which is available around the clock, 24x7. We understand that your coding assignments may have tight deadlines or that you might need assistance at any time, day or night. That's why we've made it a priority to ensure our support team is always ready to assist you. Here are the means through which you can easily contact our 24x7 customer support team:
- Live Chat: You can engage with our customer support team instantly via live chat on our website for quick assistance.
- Email: Feel free to send us an email at [email protected] for detailed queries or information.
- Phone: You can call our customer support team directly using the provided phone number on our website.
- Contact Form: Use the contact form on our website to submit your questions or requests, and our team will respond promptly.
- Social Media Platforms: We are active on social media platforms like Facebook, Twitter, and Instagram. You can connect with us through private messages or comments on our posts for inquiries, updates, or assistance. Our social media profiles are easily accessible through our website.
Our Stellar Record
Well-researched blogs to fine-tune your coding skills.
Stay informed and engaged with the ever-evolving world of coding and programming through our blog section. Here, you'll discover a wealth of knowledge, including the latest trends, valuable tips, and insightful articles on a wide range of programming topics. Our blog serves as a valuable resource for students and coding enthusiasts alike, offering tutorials and guides to expand your coding knowledge and enhance your skills. Explore our blog regularly to stay ahead in your coding endeavors and keep up with the rapidly changing tech landscape.
We Are Associated with Skilled Programming Assignment Experts
Our coding experts are the backbone of CodingAssignmentHelp.com. With years of practical experience and a deep knowledge of various programming languages and domains, they form a formidable team that ensures your coding assignments are handled with precision and expertise. They work tirelessly to deliver clean, efficient, and well-documented code, helping you not only complete your assignments but also understand the underlying concepts and best practices. Rest assured that our experts are here to guide you toward success in your programming coursework.

Average rating on 1122 reviews 4.9/5

Average rating on 973 reviews 4.9/5

Average rating on 1051 reviews 4.9/5
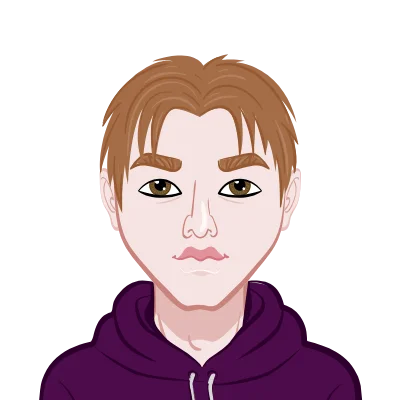
Average rating on 865 reviews 4.8/5
Read What our Esteemed Clients Think About Us
Explore what our clients have to say about their experiences with CodingAssignmentHelp.com. We take immense pride in our track record of satisfied students who have greatly benefited from our coding assignment assistance. By reading their honest testimonials, you can gain insights into how our services have made a positive impact on their academic journeys. These reviews provide a glimpse into the quality, reliability, and effectiveness of our coding support, reaffirming our commitment to helping you achieve your academic goals.
Access Our Repository of Error-Free Coding Assignment Samples
Get a firsthand look at the quality and expertise we offer through our sample assignments. These examples demonstrate our team's ability to provide well-structured, efficient, and comprehensible coding solutions. By exploring our sample assignments, you can witness the depth of our coding proficiency and how we can assist you in achieving your coding goals. Whether you need assistance with Java, Python, C++, or any other programming language, these samples showcase our commitment to delivering high-quality coding solutions for your academic success.
Frequently Asked Questions
Our FAQs have been carefully crafted to offer clear and concise answers to some of the most frequently asked questions, ensuring that users can quickly find the information they need. They serve as a valuable resource to demystify the process of availing our coding assignment help service, making it easier for users to navigate and understand our offerings. By addressing common queries upfront, we aim to eliminate confusion and provide a seamless experience for individuals seeking assistance with their coding assignments.

CMS board gets some pushback in first round of student assignment

The Charlotte-Mecklenburg Schools board will vote later this month on student assignment changes that largely impact magnet schools. Tuesday night a few dozen parents and students signed up to weigh in.
Several students cautioned the board against adding 9 th and 10 th grades to high schools located on four of Central Piedmont Community College’s campuses that offer tuition-free college credit.
“If you change the middle college program to early colleges only, having students enter in 9th grade, you'll be forcing students who are barely even teenagers to choose a career path. These students are not mature enough,” said Khai Mathlage, who attends a middle college program on CPCC’s Merancas campus.
Several parents also urged the board against moving Dorothy J. Vaughan Academy of Technology in northeast Charlotte eight miles away to Parkside Elementary, which has a newer building and plenty of room.
“What I know is the fact that the little brown boys and girls who are sitting there, who are looking at themselves to be in science, to be in technology, they’re losing their one opportunity to do that,” said Renarda Hamilton.
The plan would also switch the neighborhood school assignments for about 800 students. Parents of students at Dilworth Elementary said the board should agree to delay shifting about 100 Marie G. Davis students to Dilworth’s attendance zone until a new, larger school opens in two years.
“We're overcrowded. Most specials are being taught from carts. The art classroom…is on the gymnasium stage. There isn't enough parking for our staff,” said Lauren Scarboro, who has two children at Dilworth Elementary.
That alternative would also delay relocating a Montessori program for grades 7-12 at J.T. Williams to Marie G. Davis.
The board expects to vote on these changes Sept. 24. They only impact 1% of CMS students, but a deeper dive into student assignment will take place in the coming months.
Sign up for our Education Newsletter
- Education News written by WFAE reporter Ann Doss Helms (Mondays)
- html
- text

C-Pack of IPAs: A C90 Program Benchmark of Introductory Programming Assignments
New citation alert added.
This alert has been successfully added and will be sent to:
You will be notified whenever a record that you have chosen has been cited.
To manage your alert preferences, click on the button below.
New Citation Alert!
Please log in to your account
Information & Contributors
Bibliometrics & citations, view options, index terms.
Applied computing
Computer-assisted instruction
Computing methodologies
Machine learning
Theory of computation
Semantics and reasoning
Program reasoning
Program analysis
Program semantics
Recommendations
Multipas: applying program transformations to introductory programming assignments for data augmentation.
There has been a growing interest, over the last few years, in the topic of automated program repair applied to fixing introductory programming assignments (IPAs). However, the datasets of IPAs publicly available tend to be small and with no valuable ...
Automated clustering and program repair for introductory programming assignments
Providing feedback on programming assignments is a tedious task for the instructor, and even impossible in large Massive Open Online Courses with thousands of students. Previous research has suggested that program repair techniques can be used to ...
Information
Published in.
Hitachi, Japan
Concordia University, Canada
University College London, United Kingdom
UT Austin, United States of America
- SIGSOFT: ACM Special Interest Group on Software Engineering
In-Cooperation
- Faculty of Engineering of University of Porto
Association for Computing Machinery
New York, NY, United States
Publication History
Check for updates, author tags.
- introductory programming assignments
- programming education
- automated program repair
- semantic program repair
- syntactic program repair
- computer-aided education
- Research-article
Funding Sources
- Fundação para a Ciência e Tecnologia FCT
- European Regional Development Fund
- MEYS, ERC CZ project POSTMAN no.~LL1902
- Horizon 2020 research and innovation program
Upcoming Conference
Contributors, other metrics, bibliometrics, article metrics.
- 0 Total Citations
- 0 Total Downloads
- Downloads (Last 12 months) 0
- Downloads (Last 6 weeks) 0
View options
View or Download as a PDF file.
View online with eReader .
Login options
Check if you have access through your login credentials or your institution to get full access on this article.
Full Access
Share this publication link.
Copying failed.
Share on social media
Affiliations, export citations.
- Please download or close your previous search result export first before starting a new bulk export. Preview is not available. By clicking download, a status dialog will open to start the export process. The process may take a few minutes but once it finishes a file will be downloadable from your browser. You may continue to browse the DL while the export process is in progress. Download
- Download citation
- Copy citation
We are preparing your search results for download ...
We will inform you here when the file is ready.
Your file of search results citations is now ready.
Your search export query has expired. Please try again.

IMAGES
VIDEO
COMMENTS
Ashwin Joy. I'm the face behind Pythonista Planet. I learned my first programming language back in 2015. Ever since then, I've been learning programming and immersing myself in technology.
These free exercises are nothing but Python assignments for the practice where you need to solve different programs and challenges. All exercises are tested on Python 3. Each exercise has 10-20 Questions. The solution is provided for every question. These Python programming exercises are suitable for all Python developers.
The programming assignments are evaluated using a sophisticated autograder that provides detailed feedback about style, correctness, and (sometimes) efficiency. Which Java programming environment will I use? We supply a streamlined version of IntelliJ that is easy to install and suitable for novices. However, you are free to use any programming ...
There are 7 modules in this course. This course aims to teach everyone the basics of programming computers using Python. We cover the basics of how one constructs a program from a series of simple instructions in Python. The course has no pre-requisites and avoids all but the simplest mathematics. Anyone with moderate computer experience should ...
Programming assignments. Creative programming assignments that we have used at Princeton. You can explore these resources via the sidebar at left. Introduction to Programming in Java. Our textbook Introduction to Programming in Java [ Amazon · Pearson · InformIT] is an interdisciplinary approach to the traditional CS1 curriculum with Java. We ...
Whereas CS50x itself focuses on computer science more generally as well as programming with C, Python, SQL, and JavaScript, this course, aka CS50P, is entirely focused on programming with Python. You can take CS50P before CS50x, during CS50x, or after CS50x. But for an introduction to computer science itself, you should still take CS50x!
Programming Assignments with Examples. pdf. 139 kB 6.100L Finger Exercises Lecture 01 Solutions. pdf. 145 kB 6.100L Finger Exercises Lecture 02 Solutions. pdf. 142 kB 6.100L Finger Exercises Lecture 03 Solutions. pdf. 140 kB 6.100L Finger Exercises Lecture 04 Solutions ...
6.0001 Introduction to Computer Science and Programming in Python is intended for students with little or no programming experience. It aims to provide students with an understanding of the role computation can play in solving problems and to help students, regardless of their major, feel justifiably confident of their ability to write small programs that allow them to accomplish useful goals.
In this course, you will be introduced to foundational programming skills with basic Python Syntax. You'll learn how to use code to solve problems. You'll dive deep into the Python ecosystem and learn popular modules, libraries and tools for Python. You'll also get hands-on with objects, classes and methods in Python, and utilize ...
C is a general-purpose, imperative computer programming language, supporting structured programming, lexical variable scope and recursion, while a static type system prevents many unintended operations. C was originally developed by Dennis Ritchie between 1969 and 1973 at Bell Labs.
All Examples. Files. Python Program to Print Hello world! Python Program to Add Two Numbers. Python Program to Find the Square Root. Python Program to Calculate the Area of a Triangle. Python Program to Solve Quadratic Equation. Python Program to Swap Two Variables. Python Program to Generate a Random Number.
The best way we learn anything is by practice and exercise questions. Here you have the opportunity to practice the Java programming language concepts by solving the exercises starting from basic to more complex exercises. A sample solution is provided for each exercise. It is recommended to do these exercises by yourself first before checking ...
Programming assignments will use one of two submission methods: Script submission: Run your code in your local coding environment, then enter the submission token to complete the assignment. Web submission: Follow the on-screen steps to upload your files. Programming assignments may include multiple coding tasks for you to complete.
Creative Programming Assignments. Below are links to a number of creative programming assignments that we've used at Princeton. Some are from COS 126: Introduction to Computer Science; others are from COS 226: Data Structures and Algorithms.The main focus is on scientific, commercial, and recreational applications.
Here are 10 basic coding projects for beginners: 1. Build a chess game. Building a chess game is a great way to hone your ability to think like a developer. It'll also allow you to practice using algorithms, as you'll have to create not only the board and game pieces but also the specific moves that each piece can make. 2.
Learn C programming with interactive exercises and examples. Test your skills and improve your knowledge of C syntax, functions, pointers, and more.
Then, choose ' Programming ' or ' Computer science ' in the ' Subject area ' field and specify the number of problems/assignments you need to be done. Set the deadline. Small assignments can be completed as fast as just 24 hours. Save 11% on your first order with coupon code "WOWSALE". Give as detailed instructions on how you need ...
Simple, No-frills Programming assignment help and tutoring Service, Just submit your problem / Homework -> Get the help. Best Programming help with modest pricing. No fumbling around the expert profiles yourself, No complex forms or hourly rates. Option to solve your programming assignment 1:1 online with an expert—because growth happens when ...
Access to lectures and assignments depends on your type of enrollment. If you take a course in audit mode, you will be able to see most course materials for free. To access graded assignments and to earn a Certificate, you will need to purchase the Certificate experience, during or after your audit. If you don't see the audit option:
Can't submit programming assignment. If you're having trouble submitting a programming assignment, try the following steps: Make sure you saved your files in the correct format. To change the format, re-save your files. Decrease the size of the files, if possible. Clear your browser's cookies and cache. Making sure any ad-blocking software is ...
How our service works. Follow these four steps to get your programming assignment done. Place an order with all the requirements we have to follow. Pay for your order safely in just a few clicks. Track the progress of your task on your personal order page. Get your solution and check if it needs any free fixes.
Courses in computer science (CS) often assess student programming assignments manually, with the intent of providing in-depth feedback to each student regarding correctness, style, efficiency, and other quality attributes. As class sizes increase, however, it is hard to provide detailed feedback consistently, especially when multiple assessors ...
Get Professional Help With Any Programming Assignment. Struggling with coding? We can help with anything concerning it: from programming tasks and papers of any type, level, and complexity to academic tests and useful tips. Request a quote. Live chat. about.css. assignment.js. assignment.py. 01.assignment-instructions.
Unparalleled Programming Assignment Help Provided by Tech-Savvy Professionals. Our unwavering commitment lies in assisting students in crafting clean, comprehensible, and effortlessly executable code. With over a decade of trusted expertise in providing programming assignment help to clients worldwide, we stand as a beacon of reliability.
The development of programming education has given rise to automated program repair techniques tailored for introductory programming assignments (IPAs). Despite the promising performance of mainstream automated feedback generation systems, they still struggle to handle scenarios where there is "no matching control-flow to generate repair" well ...
The problem of automated feedback generation for introductory programming assignments (IPAs) has attracted significant attention with the increasing demand for programming education. While existing approaches, like Refactory , that employ the "block-by-block" repair strategy have produced promising results, they suffer from two limitations.
That alternative would also delay relocating a Montessori program for grades 7-12 at J.T. Williams to Marie G. Davis. The board expects to vote on these changes Sept. 24.
Pedro Orvalho, Mikolás Janota, and Vasco Manquinho. 2022. MultIPAs: Applying Program Transformations to Introductory Programming Assignments for Data Augmentation. In Proceedings of the 30th ACM Joint European Software Engineering Conference and Symposium on the Foundations of Software Engineering, ESEC/FSE 2022. ACM, Singapore, 1657--1661.